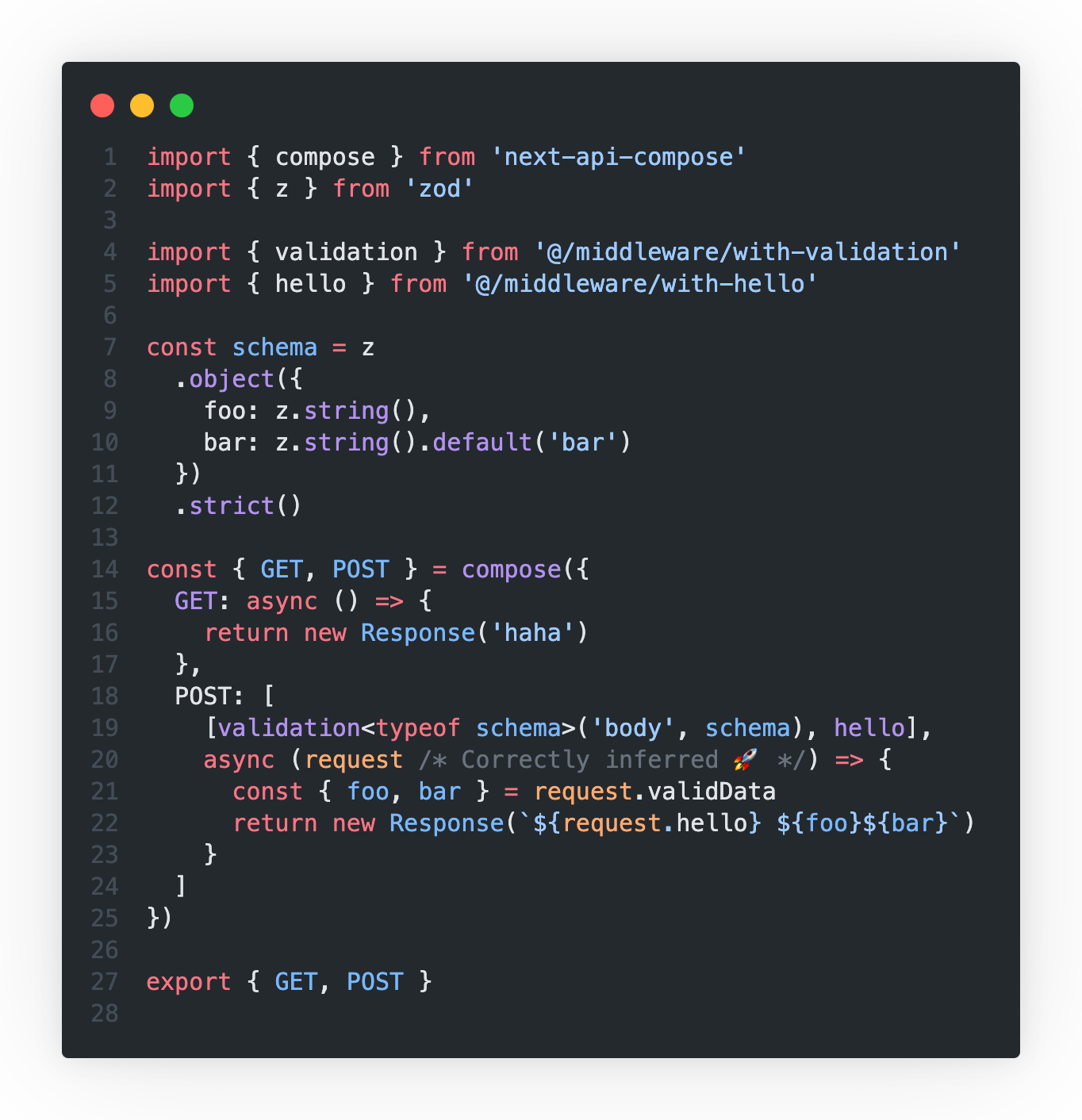
This library provides a hassle-free way of composing multiple middleware functions into one Next.js API Route Handler's method in the App Directory router.
Important
The 2.0.0
version of the library supports both app and pages directory oriented API utilities. If you're still using Pages Router and you want to migrate from versions below 2.0.0
, please read migration guide and ocassionally consider checking out intro to the App Router.
- 😇 Simple and powerful API
- 🚀 Designed both for Pages Router and App Router
- 🧪 Production-ready. 100% test coverage, even type inference is tested!
- 🥷 Excellent TypeScript support
- 🧬 Maintaining order of middleware chain
- 📦 No dependencies, small footprint
Install the package by running:
npm i next-api-compose -S
# or
yarn add next-api-compose
# or
pnpm i next-api-compose
then create an API Route Handler in the App Directory:
in TypeScript (recommended)
import type { NextRequest } from "next/server";
import { compose } from "next-api-compose";
function someMiddleware(request: NextRequest & { foo: string }) {
request.foo = "bar";
}
const { GET } = compose({
GET: [
[someMiddleware],
(request /* This is automatically inferred */) => {
return new Response(request.foo);
// ^ (property) foo: string - autocomplete works here
},
],
});
export { GET };
in JavaScript:
import { compose } from "next-api-compose";
function someMiddleware(request) {
request.foo = "bar";
}
const { GET } = compose({
GET: [
[someMiddleware],
(request) => {
return new Response(request.foo);
},
],
});
export { GET };
Handling errors both in middleware and in the main handler is as simple as providing sharedErrorHandler
to the compose
function's second parameter (a.k.a compose settings). Main goal of the shared error handler is to provide clear and easy way to e.g. send the error metadata to Sentry or other error tracking service.
By default, shared error handler looks like this:
sharedErrorHandler: {
handler: undefined;
// ^^^^ This is the handler function. By default there is no handler, so the error is being just thrown.
includeRouteHandler: false;
// ^^^^^^^^^^^^^^^^ This toggles whether the route handler itself should be included in a error handled area.
// By default only middlewares are being caught by the sharedErrorHandler
}
... and some usage example:
// [...]
function errorMiddleware() {
throw new Error("foo");
}
const { GET } = compose(
{
GET: [
[errorMiddleware],
() => {
// Unreachable code due to errorMiddleware throwing an error and halting the chain
return new Response(JSON.stringify({ foo: "bar" }));
},
],
},
{
sharedErrorHandler: {
handler: (_method, error) => {
return new Response(JSON.stringify({ error: error.message }), {
status: 500,
});
},
},
}
);
// [...]
will return {"error": "foo"}
along with 500
status code instead of throwing an error.
-
Unfortunately there is no way to dynamically export named ESModules (or at least I did not find a way) so you have to use
export { GET, POST }
syntax instead of something likeexport compose(...)
if you're composing GET and POST methods :( -
Middleware is executed as specified in the per-method array, so if you want to execute middleware in a specific order, you have to be careful about it. Early returned
new Response()
halts the middleware chain.
Igor 💻 |
Maksymilian Grabka |
kacper3123 📖 |
The project is licensed under The MIT License. Thanks for all the contributions! Feel free to open an issue or a pull request even if it is just a question 🙌